Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Java Loops And Controls
Most of the programming languages have loop controls and conditions in code, let’s try to understand java loops and controls.
Java Loops are repetitive blocks where any code or statement will be executed multiple times until the end condition of loop is met.
Diagram of Java Loops Controls:
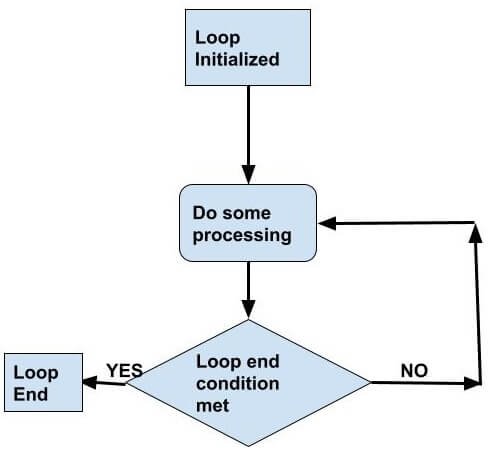
Below are 4 types java loops controls:
- for – for loop is used to repetitively execute a block of code until the end condition of for loop met.
- foreach – foreach is similar to for loop because it also rules repetitively but foreach is available in collection classes for example List class where a list element can be repeatedly processed till the end of list reaches.
- while – as the name suggests it is a loop but with while which means while certain condition is true/met the loop will continue.
- do .. while – it is similar to while loop only difference is in while loop it will first check condition and then start execution of statements inside loop, so if condition is no met at start, it may never execute any code in loop and skip it but with do while first it will execute the code and then check if condition is met or not, so at least once the loop will execute for sure.
Now let’s see more details and examples of each type of loop in java:
Java For Loop
Java for loops runs and executes code inside it as long as the end condition of the loop is not met.
Below are two different types and syntax of for loop:
Different Syntaxes of for loop:
For loop type 1:
for(variable name = start value; condition to end loop; increase variable value) {
//Some code here which will run in loop
}
For loop type 2
for(variable name with type: List Of Data) {
//Some code here which will run in loop
}
Example of two types of for loop:
public class Loops {
public static void main(String[] args) {
//for loop between 1-10 for(int i=1; i<=5; i++) { System.out.println("in for loop value of i="+i); }
//Another for loop which runs in array of data int[] arrayOfInt = {1, 2, 3, 4, 5}; for(int i : arrayOfInt) { System.out.println("other for loop value of i="+i); } } }Â
Below is the output of above program:
in for loop value of i=1
in for loop value of i=2
in for loop value of i=3
in for loop value of i=4
in for loop value of i=5
other for loop value of i=1
other for loop value of i=2
other for loop value of i=3
other for loop value of i=4
other for loop value of i=5
Java foreach Loop
Java foreach loop runs on a list or a collection type data, it is used to reach each element in aa list and then we can write our code to process the data.
Syntax of foreach loop:
.foreach(data => some operation/code)
Example of foreach loop:
Â
import java.util.ArrayList; import java.util.List; public class Loops { public static void main(String[] args) { //List of integer created List listOfInt = new ArrayList<Integer>(); listOfInt.add(1); listOfInt.add(2); listOfInt.add(3); listOfInt.add(4); listOfInt.add(5); //foreach loop on list of integer, this is written with lambda expression see that arrow between data and print statement listOfInt.forEach(data -> System.out.println("in java foreach loop i="+data)); } }
Below is the output of above program:
in for loop value of i=1
in for loop value of i=2
in for loop value of i=3
in for loop value of i=4
in for loop value of i=5
other for loop value of i=1
other for loop value of i=2
other for loop value of i=3
other for loop value of i=4
other for loop value of i=5
Java foreach Loop
Java foreach loop runs on a list or a collection type data, it is used to reach each element in aa list and then we can write our code to process the data.
Syntax of foreach loop:
.foreach(data => some operation/code)
Example of foreach loop:
import java.util.ArrayList; import java.util.List; public class Loops { public static void main(String[] args) { //List of integer created List listOfInt = new ArrayList<Integer>(); listOfInt.add(1); listOfInt.add(2); listOfInt.add(3); listOfInt.add(4); listOfInt.add(5); //foreach loop on list of integer, this is written with lambda expression see that arrow between data and print statement listOfInt.forEach(data -> System.out.println("in java foreach loop i="+data)); } }
Output of above foreach loop program:
in java foreach loop i=1 in java foreach loop i=2 in java foreach loop i=3 in java foreach loop i=4 in java foreach loop i=5
Java While Loop
Java while loop runs as long as long as the while condition is true.
Syntax:
while(){
//some code to be executed in loop
}
Examples of java while loop:
public class Loops { public static void main(String[] args) { //while loop example int i=1; while(i<=5) { //In this block you recitative code will run System.out.println("in while loop i ="+i); i++; } } }
Output of above while loop program:
in while loop i =1 in while loop i =2 in while loop i =3 in while loop i =4 in while loop i =5
Java Do While Loop
As we discussed previously that do while is the same as while loop, but it ensures that it will run once before checking end condition, so even if end conditions are always true, it will execute your code once before exiting the loop.
Example of Java Do While Loop:
Output of Above Java Do While Loop Program:
in do while loop i =10
See the difference between while and do while loop in above programs, only it printed the value of i=10 and exited the loop because end condition met immediately, i<=5 where i=10 so condition 10<=5 will return false and hence loop ended.
Java Loop Continue and Break Statement
In loops we can use continue statements to continue the loop or can use break to break and exit the loop.
What is the use of continue and break in java?
In a loop if you were looking for data and you already found it don’t want to continue the loop and waste resources you can simply add a break statement and exit from the loop before it completes.
Similarly if you want to continue the loop and don’t want to process lines below continue in some cases then you can use continue statement to continue the loop and skip remaining lines in loop.
Example of break:
public class Loops { public static void main(String[] args) { //for loop between 1-10 for (int i = 1; i <= 5; i++) { if (i == 4) { System.out.println("break condition, we will exit the loop i=" + i); break; } System.out.println("in for loop checking on break i=" + i); } } }
Output:
in for loop checking on break i=1 in for loop checking on break i=2 in for loop checking on break i=3 break condition, we will exit the loop i=4
Example of continue:
public class Loops {
public static void main(String[] args) {
//for loop between 1-10
for (int i = 1; i <= 5; i++) {
if (i == 4) {
System.out.println("In continue condition, we will not check next code and continue the loop i="+i);
continue;
}
System.out.println("In for loop checking on break i="+i);
}
}
}
Output:
In for loop checking on break i=1 In for loop checking on break i=2 In for loop checking on break i=3 In continue condition, we will not check next code and continue the loop i=4 In for loop checking on break i=5