Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Top 50 Java Interview Questions and Answers
Below are top 50 Java Interview Questions and their answer of 2021, these questions cover core java, some collections and OOPS concepts of Java.
Q1. What is JDK, JRE and JVM ?
JDK: JDK stands for Java development kit, it is a software development kit to compile and run java.JDK has JRE within it and JRE contains JVM when it executes within it.
JRE: JRE stands for java runtime environment, it comes within JDK tool kit package and also can be downloaded separately, it is used to run any java application, when it executes JVM gets created and all java programs run within JVM.
JVM: JVM stands for java virtual machine. It is a running process where any java program executes. JVM is used to run any JVM based programming languages code for example Java, Scala etc. When we run a java command and execute the class file using it then JVM gets created.
Q2. What is public static void main(String args[]) in Java.
main() method in java is an entry point in a java program from where java starts execution code. This method is public because it needs to be accessible publicly.
Let’s see different components of main() method:
public – It is a keyword which specifies access level of method.
static – It is a keyword which makes method static in nature and can be accessed at class level without need to any instance.
void – return type which means this method will not return anything.
main() – name of method
String args[] – It is an argument that main method can take, so main can take an array of strings.
Q3. Why is Java called platform independent?
Java compiler generates bytecodes (.class file) from .java program files which can be executed in any machine or OS regardless of their architecture as long as JRE is available in that machine and so because of its ability to run in any machine or OS it is called platform independent.
Q4. Why is Java not a 100% object oriented programming language?
Java is not 100% OOP language because it still has 8 primitive data types in use which are not Class or Object, these are int, float, double, long, short, boolean, byte and char.
Q5. What are primitive and non primitive(Wrapper) in Java?
Primitive is a data type int in java, primitive data types are int, float, double, long, short, boolean, byte and char.
Non-primitive or wrapper classes are classes which wrap or hold primitive data types in a class and objects can be created from them, examples of primitive data types are Float, Integer and Boolean etc.
Q6. What is a constructor in java, tell us about types of constructor and default constructor in java?
Constructor: In java class there is a block of code which looks like a method but it has the same name as class and is used to initialize the object of class is called constructor.
There are two types of constructors:
1. Parameterized constructor: Constructors which take one more than one parameter are called parameterized constructors.
2. No argument or non parameterized constructors: Constructor without any parameter are called non parameterized constructors.
Default constructor: Each class in java has a default which we don’t need to declare and whenever we create an object without arguments this is called. We may choose to override its definition and write our own as well.
Q7. What is a singleton class and how can we make a class singleton?
Singleton is a class which has a single(one) instance(object) in the entire JVM, this can be achieved by creating a class with private default constructor and logically creating one instance and returning the same instance each time whenever accessed by accessing any static method of the class.
Q8. ArraList vs Vector What are the differences?
Below are the main difference between array list and vector in java:
1. ArrayList is not synchronized and not thread safe and vector is synchronized and thread safe.
2. Array list operations are fast because they are not synchronized and vector operations are slow because they are thread safe.
3. ArrayList increases its size by 50% when about to get full but vectors doubles its capacity when about to get full.
4. Itrator is used to iterate ArrayList while vector uses iterator and enumerator both for iterating the elements.
Q9. equals() vs == in Java, what is the difference?
equals() method is used to compare the value or content of the object == is used to compare the memory address of two objects. A simple example can be two string objects with the same value equals will return true but == will return false as it has a different address.
Example:
public class Main {
public static void main(String[] args) {
String greeting = new String("Hello");
String greeting1 = new String("Hello");
System.out.println(greeting==greeting1);
System.out.println(greeting.equals(greeting1));
}
}
O/P:
false
true
Q10. Heap vs Stack memory in Java?
Below are differences between Stack and Heap memory in Java:
1. Stack memory is used by thread, each thread has its own stack memory while heap memory is used by the entire JVM/application and stores objects.
2. Stack memory area can’t be accessed by other threads while objects in heap memory are globally accessible.
3. As the name suggests it is a stack and follows LIFO(Last in first out) manner to free memory while objects in heap memory are cleaned by GC when no more needed.
4. It exists until thread is executing while heap exists for the entire application life and gets cleaned by GC based on its algorithm.
Q11. What is a package and its benefits in java?
Package is similar to folder structure which may be single or hierarchical where source code and classes will be available, this gives ability to create package or space for similar type of classes, for example java.util package in java holds a lot of utility classes in it.
Some benefits:
· It provides the ability to package similar classes in one place.
· If there are two classes with the same name they can live in different packages, which will cause name clashes.
· Provides modularity and easy maintenance.
· It may be used to create hierarchical packages for example com.business package may contain business related class and com.business.details may contain more detail classes related to business.
Q12. Why are pointers not used in Java?
Pointers are used to directly access memory location or address which used to cause a lot of problem in C programming, also it may increase security risk, this is the reason to avoid direct memory access it is not used in Java, in addition to this JVM is responsible for memory management in Java, if we will have pointer will cause conflicts and problem.
Q13. What is JIT (Just in time) compiler in Java?
JIT compile is used to convert the small piece of bytecode into machine instruction at run time whenever JVM asks for it, this avoids conversion of huge chunk of bytecodes and instead only small part of code (method etc.) gets converted from bytecode to machine instructions and sent to processor.
Q14. Please tell us about access modifiers and their types in Java?
Access modifiers are java reserved keywords used to restrict access of a class and its members to another class or packages.
They are of 4 types:
1. Default – Allows access within the package.
2. Private – Allows access in the same class only.
3. Protected – Allows access within package and subclass outside the package..
4. Public – Accessible everywhere.
Q15. What is a class with an example?
A class is a blueprint or skeleton of real world objects which has different methods and variables as members which define its behaviour.
Example:
public class Tree { //Class
int age = 100; //Variable
void giveOxyzen() { // a method in class
}
}
Q16. What is an object and how to create an object?
Object is an instance of a class or real life objects, for example if we have a class named Tree(Blue print) and from that class we create one instance of tree lets say tree1 then it will be called object of class.
Objects can be created using new keyword and with the name of Class.
Example:
MyClassName myClassObject = new MyClassName()
In this example we MyClassName is a class and myClassObject is an object of class. There are other techniques to create objects as well for example cloning, serialization etc.
Q17. Please tell us about Object Oriented Programming (OOP)?
Object oriented programming is based on the concept of Object and Class, everything in OOP is an object and by using those objects entire program logic is created and executed.
This has resemblance to real world objects for example Tree, Car, House etc. which we can define as a class and create objects of them and use to create complex logic.
Q18. Tell us about OOPs and different concepts of OOP in Java?
OOPs stands for object oriented programming which is based on objects and class, below are 4 important concepts of OOPs in Java:
1. Inheritance: Inheritance means inheriting properties of another class (parent/super) class by another child(sub) class.
2. Encapsulation: It means encapsulating the data in a single unit and exposing it with the help of getter and setter methods, for example a class with private variables and a getter and setter public method for those variables.
3. Abstraction: Creating a layer or abstraction between definition and actual implementation of behaviour(methods), example java abstract classes and their implementation.
4. Polymorphism: Polymorphism means ability to take multiple forms. For example, a method with the same name and different number parameters can be written many times with different functionality in a class will be called polymorphism.
Q19. Tell us about local and an instance variable and their differences?
A local variable is such variable which is declared and used within scope of a block or method while an instance variable is declared within scope of an object at class level which can be used across class by object.
In the below example of Tree class int age is instance variable and oxyMethod String is local.
Example:
public class Tree {
int age = 100; //Instance variable
void giveOxyzen() {
String oxyMethod = “I am oxy method”; //Local variable
}
}
Q20. What are differences between methods and constructors in Java?
Below are differences between methods and contractors:
1. Methods are used to define behavior of class or object while constructors are used to initialize and create an object of class.
2. Methods may or may not have the same name as class but constructors always have the same name as class.
3. Methods always have return type but constructors don’t and they don’t return anything.
4. Methods need to be invoked by the object while constructors get initialized when the object is created.
5. Methods can be executed many times with the help of the same object while constructors can be initialized once while creating the object.
Q21. What are final, finally and finalize()?
final: It is a keyword used in java to make nature of class and their attributes final, for example a class with final keyword can’t be extended by another class, similarly a method with final keyword can’t be overridden or changed, similarly a variable with final keyword can’t does not allow further change in its value once initialize.
finally: finally is used in exception handling with try and catch block, if we want to run some critical code regardless of exception or no exception in try block code then we can write in finally block.
finalize(): finalize() method is invoked by GC when it determines that object is no more referred or used to claim the resources, we can call this explicitly on any object object.finalize() but it won’t destroy the object until GC runs.
Q22. What are the differences between break and continue statements?
Below are main differences between break and continue:
1. Break is used in switch and loop in java while continue is only used in loops.
2. As soon as it is called the loop or switch will break execution and go out of the current loop while continue moves execution to the next iteration of the loop.
3. Break terminates the current loop, if there are loops within the loop then it will break current and pass control to the outer loop while continue continues within the same loops.
Q23.What is an infinite loop in Java? Explain with an example?
If the end conditions of a loop are written in such a way that it will always return true and continue the loop then it is called infinite loop, we should never write such loops unless we really know what we are doing.
Example 1: Infinite while loop
while(true) {
}
Example 2: Infinite for loop
for(;;) {
}
Q24. Please explain the difference between this and super() in Java?
Below are main difference between this and super()
- this is a keyword which refers to current instance of object while super() refers to current instance of parent class.
- this is used to call default constructor of same class while super is used to call parent class constructor.
- this is used to access current class method and variables while is used to access parent class method and variables.
Q25. What is Java String Pool?
String pool is a memory area in java heap where all strings are stored, if a new String object is created it will check in pool, if it already exists then it won’t create a new object but will point to previously created one else a new object will be created.
Example:
String str1 = “Hello”;
String str2 = “Hello”;
String str3 = “Namaste”;
If we do str1 == str2, it will give true and if we do str1 == str3 it will give false.
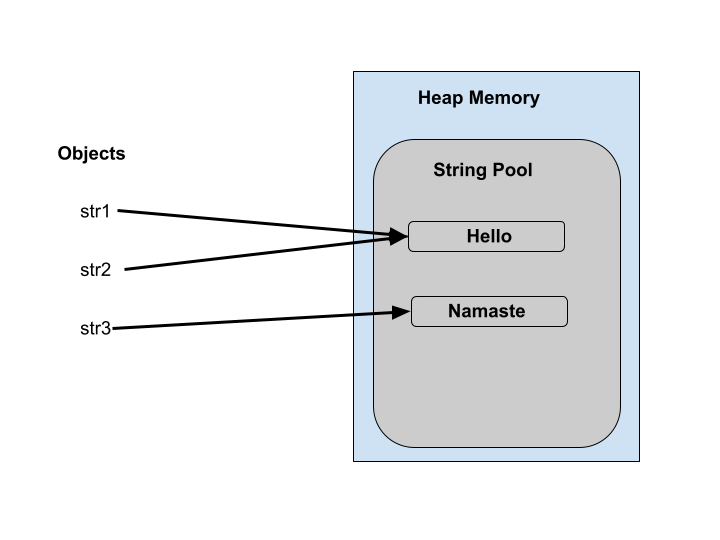
Q26. Please tell us the difference between static and non-static methods in Java.
Below are differences between Static Method and Non-Static Methods:
- static keyword is used before method name while in non static method no use of any static keyword before method name.
- static method can be accessed by class name without creating an instance of class while in non static method can be accessed with the help of an object of class.
- A static method/block can’t access non-static static while a non static method can access non static and static methods and variables.
Q27. What is constructor chaining in Java?
Constructor chaining is invoking one constructor from another, for example if there is a super class and sub class and if we add super() inside sub class then it will first initialize super/parent class constructor and then sub class. We can use this() and super() for creating constructor chains in java.
Q28. Please tell us the difference between String, StringBuilder, and StringBuffer.
Property | String | StringBuilder | StringBuffer |
Memory | Inside String pool in Heap memory | Heap Area | Heap Area |
Mutability or change of state | Immutable | Mutable | Mutable |
Thread Safe | Yes | No | Yes |
Performance | Efficient | Fast | Slow |
Q29. Explain class loader in JVM?
Class loaders reside inside the JVM and load the classes based on requirement, there are three types of class loader
1. Bootstrap Class Loader: It loads key core library classes rt.jar etc which helps in loading other classes.
2. Extension Class Loader: It loads key extension classes found at $JAVA_HOME/lib/ext
3. System/Application Class Loader: It loads application’s classes.
Q30. Explain string immutability and benefits of it?
String object’s values can’t be changed and as soon as we try to change or do some operation a new String object is created and hence its state can’t be mutated or changed.
This is very useful for using as a key, security, caching and in multi-threading. String objects are stored in a string pool, a special memory area within JVM’s heap memory.
Q31. Explain the differences between an array and an array list?
Array | ArrayList |
It can’t contain different types of data. | It may contain different types of data. |
While creating, size must be specified. | Size is dynamic and no need to specify. |
To access data we need to specify the index. | We can access directly without an index. |
It can hold primitive data types | It can’t hold primitive, only objects. |
Q32. What is a Map in Java?
Map is an interface in java collection which has different implementations. Maps can hold key and value pairs as elements in it and based on key we can find the value and also can change the value of the same key if we put something with the same key and different value.
Maps can’t have duplicate keys, each key will have only one value. Type of key and value can be any Class.
Q33. What is collection in Java? Give details of different collections, classes, methods in it.
Collection is a framework in java which provides different interfaces, classes and methods to create real world data structures like set, queue, list and manipulate them. Main interfaces in the collection are List, Queue and Set.
The below image shows the complete hierarchy of the Java Collection:
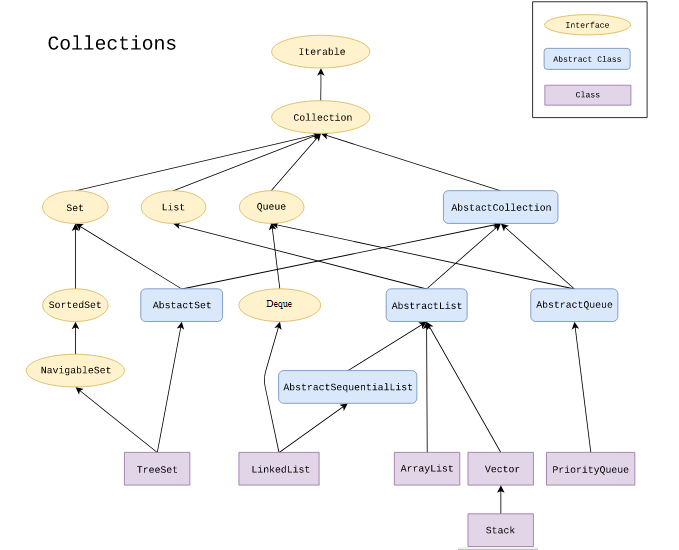
Q34. What is Polymorphism?
Polymorphism is one of 4 major concepts of OOPs in Java. Polymorphism means ability to take multiple forms, we can break polymorphism into two parts poly and morphism where poly means multiple and morphism means form. In OOP methods in a class may look the same by name but can perform different actions which will be called polymorphism. A simple example can be a method can be declared multiple times with different arguments in the same class which will have the same name but different arguments and it is an example of polymorphism.
Q35. What is runtime polymorphism or dynamic method dispatch?
Runtime polymorphism is achieved at execution or run time, if we have a class and a child class extends it and overrides its method and at runtime, if objects of child and parent created and assigned to parent type and common method called in both, then at run time it will determine which of class’s method (parent or child) to call and execute, this is called runtime polymorphism.
Example:
In below example Car class is inherited by PetrolCar and ElectricCar and displayCarType() method is available in all three classes, so when we call with help of car’s object it executes displayCarType() method of Car class and when we call same method with instance of PetrolCar will call displayCarType() of PetrolCar and same is with ElectricCar class.
Note that all instances of different classes are still assigned to only Car class.
class Car{
public void displayCarType() {
System.out.println("I am common car type.");
}
}
class PetrolCar extends Car {
@Override
public void displayCarType() {
System.out.println("I am PetrolCar.");
}
}
class ElectricCar extends Car {
@Override
public void displayCarType() {
System.out.println("I am ElectricCar.");
}
}
class DemoRunTimePolymorphisam{
public static void main(String[] args) {
Car car = new Car();
Car petrolCar = new PetrolCar();
Car eCar = new ElectricCar();
car.displayCarType();
petrolCar.displayCarType();
eCar.displayCarType();
}
}
Output
I am common car type.
I am PetrolCar.
I am ElectricCar.
Q36. What is abstraction in Java?
Abstraction is a way to hide details of actual implementation and provide abstract information of class and such class is called abstract class, the class which uses(extends) abstract class is responsible for implementation of logic of abstract class. This is called the abstraction concept of OOP in java.
Abstraction can be achieved by two ways in java:
1. By using abstract classes. (Partial abstraction)
2. By creating and implementing interface (100% abstraction)
Q37. What do you mean by an interface in Java?
An interface is similar to an abstract class or a class which will have declaration of methods to be implemented, some default and static methods and static final variables.
Java interfaces may have no methods or variable at all.
Example: Tree interface with methods not implemented.
public interface Tree {
public void getOxygen();
public void giveFruit();
public void doPhotosynthesis();
}
Q38. Please explain the difference between abstract classes and interfaces?
Abstract Class | Interfaces |
It may provide some methods already implemented. | It used to have no methods but since java 8 there can be some special methods in the interface. |
A class can extend one abstract class | A Class may implement multiple interfaces |
In abstract class we declare abstract method with abstract keyword. | In interface there is no need to use abstract keywords. |
An abstract class can have instance variables | An Interface cannot have instance variables |
An abstract class can have public, private, or protected visibility. | An Interface visibility must be public (or) none |
An abstract class can contain constructors | An Interface cannot contain constructors |
Abstract classes are fast | Interfaces are slow as it requires extra indirection to find the corresponding method in the actual class |
Q39. What is inheritance in OOPs?
Inheritance means inheriting something in OOP, if a class inherits behavior of another class it is called inheritance and class which is being inherited is called parent or super class and class which inherits parent class is called child or subclass.
By using inheritance we can bring methods and other variables to the child class from the parent based on access level and use them as they are part of the child class.
Note – Parent class is called super class and child class is called sub class in java.
Q40. What are the different types of inheritance in Java?
Below are different types of inheritance:
1) Single Inheritance – If a sub class inherits another super class is called single inheritance
Example:
class ClassA {
//Parent code
}
class ClassB extends ClassA {
//Child code
}
2) Multilevel Inheritance – If a sub class inherits one super class which inherits another super class and so on it is called multilevel inheritance
Example:
class ClassA {
//Parent code
}
class ClassB extends ClassA {
//Child code
}
class ClassC extends ClassB {
//Child code
}
3) Hierarchical Inheritance – If two classes inherit same parent class it will be called hierarchical inheritance
Example:
class ClassA {
//Parent code
}
class ClassB extends ClassA {
//Child code
}
class ClassC extends ClassA {
//Child code
}
4) Hybrid – If we mix any of the above three we can call it hybrid inheritance.
Q41. What are types of polymorphism and explain method overloading and method overriding?
Types Of Polymorphism:
There are two types of polymorphism in java as mentioned below;
· Compile Time Polymorphism – It is achieved while compile time.
· Runtime Polymorphism – It is achieved at run time.
Compile Time Polymorphism (Overloading):
Compile time polymorphism is achieved at code creation and compilation, if we have more than one method with the same name but different arguments in a class then it will be called compile time polymorphism.
Example
In the below example we create Operator class with method operate() with multiple definitions and arguments.
class Operator{
public void operate() {
System.out.println("I am no argument operator");
}
public void operate(int a) {
System.out.println(“I am single argument operator, value of a is:”+a);
}
public void operate(int a, int b) {
System.out.println(“I am two arguments operator, value of a is: “+a+” and b is: “+b);
}
public static void main(String[] args) {
Operator operator = new Operator();
operator.operate();
operator.operate(10);
operator.operate(10, 20);
}
}
Output
I am no argument operator
I am single argument operator, value of a is:10
I am two arguments operator, value of a is: 10 and b is: 20
Runtime Polymorphism (Overriding)
Runtime polymorphism is achieved at execution or run time, if we have a class and a child class extends it and overrides its method and at runtime, if objects of child and parent created and assigned to parent type and common method called in both, then at run time it will determine which of class’s method (parent or child) to call and execute, this is called runtime polymorphism.
Example:
In below example Car class is inherited by PetrolCar and ElectricCar and displayCarType() method is available in all three classes, so when we call with help of car’s object it executes displayCarType() method of Car class and when we call same method with instance of PetrolCar will call displayCarType() of PetrolCar and same is with ElectricCar class.
Note that all instances of different classes are still assigned to only the Car class.
class Car{
public void displayCarType() {
System.out.println("I am common car type.");
}
}
class PetrolCar extends Car {
@Override
public void displayCarType() {
System.out.println(“I am PetrolCar.”);
}
}
class ElectricCar extends Car {
@Override
public void displayCarType() {
System.out.println(“I am ElectricCar.”);
}
class DemoRunTimePolymorphisam{
public static void main(String[] args) {
Car car = new Car();
Car petrolCar = new PetrolCar();
Car eCar = new ElectricCar();
car.displayCarType();
petrolCar.displayCarType();
eCar.displayCarType();
}
}
Output
I am common car type.
I am PetrolCar.
I am ElectricCar.
Q42. Can you override a private or static method in Java?
It is not possible to override a private method as it is accessible to only the class in which it is defined, you may create another method in child or sub class if with the same name, similarly you can not override static method as well.
Q43. What is multiple inheritance? Is it supported by Java?
Multiple inheritance is a type of inheritance where a child or subclass can inherit properties from multiple parent classes at same time. It is not supported in java because it may lead to problems if two parent classes have the same methods and if we have to call which one will be invoked.
Q44. What is encapsulation in Java?
Encapsulation means hiding something in terms of the OOP concept. In java if we hide some variable from outside class then it will be called encapsulation. In plain words capsules are used to hide drugs(medicine) so we don’t feel bad while taking to cure any disease, not exactly but similarly encapsulation hides some variables in class.
This can be achieved by making the variable private and creating public getter and setter methods to access the variable.
Q45. What is an association?
Association is a relation between two classes. To achieve association we used objects of classes.
Association can be of between one to one, one to many, many to one and many to many objects of classes.
Q46. What do you mean by aggregation?
Aggregation is a form of association where one class has an object(s) of another class in such a way that destruction of any one of the class objects won’t destroy another, they are loosely associated. Aggregation is called has-a relation.
Example:
The Army has AirForce and AirForce has Pilot, in this example, destruction of one does not necessarily mean another can’t exist.
Q47. What is composition in Java?
Composition is a form of association where one class has object(s) of another class in such a way that destruction of first class object leads to destruction of other class object available in first class. Composition is also called part of relation where object of one class resides inside object of another class and it is part of it. In composition objects are tightly coupled.
Example:
A HumanBody class having object of class Heart is an example of composition where destruction of HumanBody object will destroy Heart object as well, Heart can’t live without HumanBody.
Q48. What is a marker interface?
Marker interface is such an interface which has no attributes or methods declared, it is an empty interface. Clonable and Serializable are such interfaces.
Example:
public interface MyCustomMarkerInterface {
}
Q49. What is shallow and object cloning in Java?
Object cloning is a technique in Java to create a copy of an object with the same values of the original object, a class must implement a cloneable interface to get cloned, it is also called shallow cloning because if there are other objects/references in class being cloned, it will still point to same object and won’t create separate copy, if new clone or original class update value of reference it will get updated.
Q50. What is a copy constructor in Java?
A copy constructor is a constructor in a class which takes its own class’s instance as a parameter and creates an instance of the same class. It can be used to do shallow cloning of classes.