Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Java Abstraction
Abstraction is a way to hide details of actual implementation and provide abstract information of class and such class is called abstract class, the class which uses(extends) abstract class is responsible for implementation of logic of abstract class. This is called abstraction concept of OOP in java.
Abstract Class
Abstract class is defined with abstract keyword in class name, few important points related to abstract class in java-
- Abstract class will always have abstract key word with class name.
- Abstract method is just definition or signature of a method but actual implementation is not provided and class which extends it will be responsible for implementation.
- Abstract class may or may not have abstract method.
- Abstract class may or may not have concrete or implemented methods.
- Abstract class can’t be instantiated.
Abstract Method
Abstract methods are defined in an abstract class with abstract keyword.
- Abstract method will always be inside an abstract class.
- Abstract method will have abstract keyword with method name.
- Abstract class and methods can’t be initialized or accessed directly, a sub class should extend it and provide implementation of all abstract methods in abstract class.
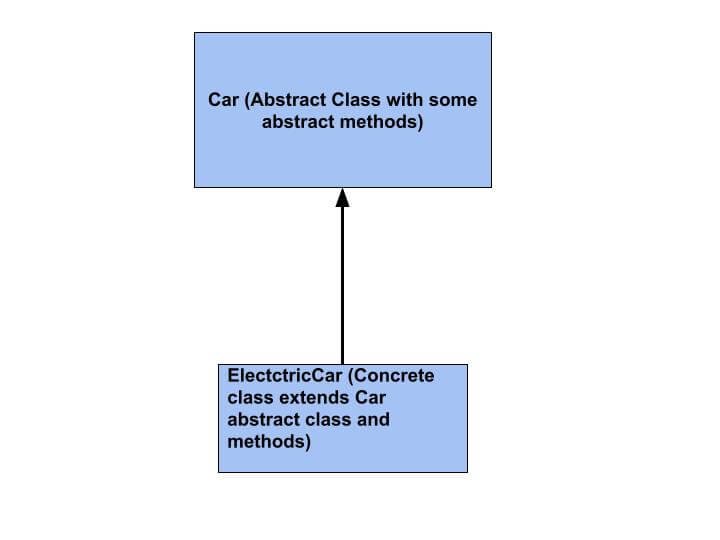
Example of Abstract Class, Method and Usage
In below code you can see different abstract classes, Car and Car1 are abstract and there is no implementation class so can’t be used or initialized, Car2 is extended and implemented by ElectricCar class and so abstract method is implemented and also already implemented abstract method is available in ElectricCar.
//Abstract class with nothing abstract class Car { } //Abstract class with one already implemented method abstract class Car1 { public void showCarName() { System.out.println("Car name is car1"); } } //Abstract class with abstract and normal methods abstract class Car2 { abstract void showCarPrize(); public void showCarName() { System.out.println("Car name is Ford"); } } class ElectricCar extends Car2 { @Override void showCarPrize() { System.out.println("eCar prize is 200000 USD"); } } class AbstractDemo { public static void main(String[] args) { Car2 eCar = new ElectricCar(); eCar.showCarName(); eCar.showCarPrize(); } }
Output:
Car name is Ford
eCar prize is 200000 USD
References
Reference article from Oracle:Â Java Abstraction