Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Java Array
Java array is wrapper class in java which is used to store same type of data in a sequence or list, for example if we want to store names of 10 people we can use string type array to hold list of name.
- Array stores multiple same type of data in an index.
- Array index starts with 0 and ends with total length of array -1.
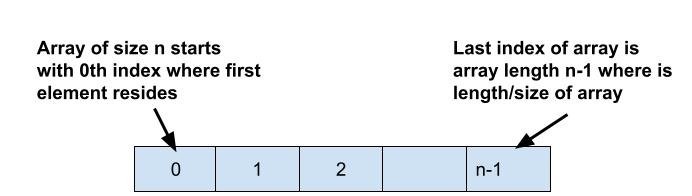
Syntaxes of Java Array
Declaration of Array
<Array type>[] <name of array>;
Initialization of Array
<array variable> = new datatype[size];
Example of Array
public class JavaArrayExample {
public static void main(String[] args) {
String[] strArray; //declaration of array
strArray = new String[5]; // Initialization of string array
strArray[0] = "John";
strArray[1] = "Meena";
strArray[2] = "Mr Tesla";
strArray[3] = "Sandy";
strArray[4] = "Rax";
System.out.println("0 index array element value = "+strArray[0]);
//declaration and initialization with size in one line
String[] strArray2 = new String[5];
}
}
Output
0 index array element value = John
Types Of Array

Single dimensional array
Single dimensional array stores one type of data element in sequence.
Syntax of Single Dimensional Array
<Array data type>[] <name of array> = new datatype[size];
Example of Single Dimensional Array
public class JavaArrayExample {
public static void main(String[] args) {
    int[] intArray = new int[3]; //single dimensional array
    intArray[0] = 10;
    intArray[1] = 11;
    intArray[2] = 12;
    System.out.println("0 index array element value = "+intArray[0]);
}
}
Output
0 index array element value = 10
Multidimensional Array
Multi-dimensional array stores one type of data element in multiple sequence.
Syntax of Multidimensional Array
[][] arrayVatiableName = new Data type[size][size];
This syntax will create two dimensional array or multidimensional array, see carefully the rectangular [] braces by using 1 we create single dimensional, by using more than 1 we create multidimensional arrays.
Example of Multidimensional Array
public class JavaArrayExample {
public static void main(String[] args) {
int[][] intArray = new int[3][3]; //multidimensional array
intArray[0][0] = 22;
intArray[0][1] = 11;
intArray[0][2] = 12;
intArray[1][0] = 10;
intArray[1][1] = 11;
intArray[1][2] = 12;
intArray[2][0] = 10;
intArray[2][1] = 11;
intArray[2][2] = 12;
System.out.println("[0][0] index array element value = "+intArray[0][0]);
}
}
Output
[0][0] index array element value = 22
For Loop On Array
We can loop through any array and access each element.
Syntax
for( variable type: array name) {
}
Example
public class JavaArrayExample {
public static void main(String[] args) {
//Another way to create array of string
String[] names = { "Rax", "John", "Tom"};
//for each loop on array
for(String name: names){
System.out.println(name);
}
}
}
Output
Rax
John
Tom
References
You may read more on oracle page: https://docs.oracle.com/javase/tutorial/java/nutsandbolts/arrays.html
Next Article To Read
You may be interested to read next articles: