Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Java Collections
Java collection is implementation of real life data structure in java along with a lot of useful methods to use them. Java collections have List, Set, Queue and Map(not directly part of collections) and many more, these data structures are implemented by creating a lot of interfaces, abstract classes and classes.
Java collections have methods like sort, min, max, search, contains etc. which can be applied on data implemented data structure classes like ArrayList, HashMap, Set etc.
Java collections is an architecture or a framework which provides a lot of built in data structure and types.
Collection Framework
- It is an architecture.
- It implements real life data structures like list, Queue, set and more.
- It provides libraries to use and manipulate these data structures.
- It has interfaces and their implementation classes.
- It implements algorithms of those data structures to do any operation.
Hierarchy of Collection
At the top of the collection interface is Iterable and then the collection interface and then other interfaces and classes come. Collection interfaces and classes are inside the “java.util” package.
See below diagram of collection interface:
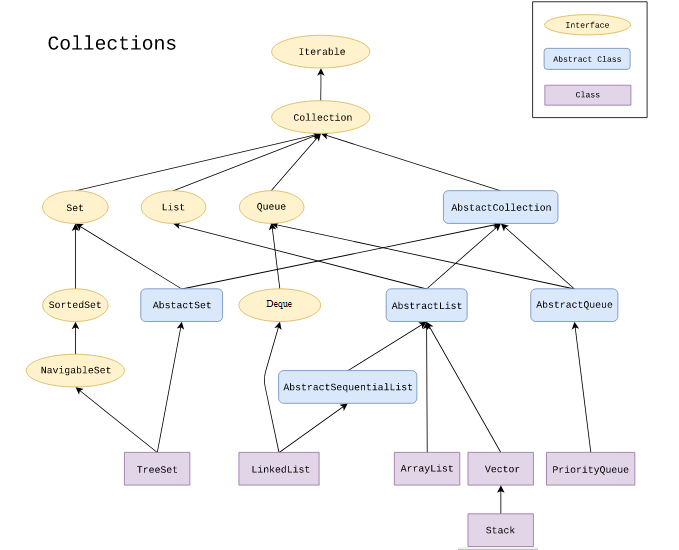
Methods of collection Interface
No. | Method | Description |
1 | public boolean add(E e) | Adds element in collection |
2 | public boolean addAll(Collection<? extends E> c) | Adds all elements of one collection in another. |
3 | public boolean remove(Object element) | Removes elements from a collection. |
4 | public boolean removeAll(Collection<?> c) | Removes all elements from a collection. |
5 | default boolean removeIf(Predicate<? super E> filter) | Conditionally removes an element. |
6 | public boolean retainAll(Collection<?> c) | It deletes all elements and keeps only specified. |
7 | public int size() | Size of collection(no of elements in collection) |
8 | public void clear() | Removed elements from collection |
9 | public boolean contains(Object element) | Returns Boolean if the collection contains a specific element. |
10 | public boolean containsAll(Collection<?> c) | Returns Boolean if the collection contains specified collections. |
11 | public Iterator iterator() | Returns integrator to integrate the collection. |
12 | public Object[] toArray() | Converts collection to array |
13 | public <T> T[] toArray(T[] a) | Converts collection to array |
14 | public boolean isEmpty() | Checks if a collection is empty or not and returns boolean. |
15 | default Stream<E> parallelStream() | Returns parallel stream of collection. |
16 | default Stream<E> stream() | Returns stream. |
17 | default Spliterator<E> spliterator() | Splits. |
18 | public boolean equals(Object element) | Checks if the collection is equal or not. |
19 | public int hashCode() | Returns hashcode (number) of collection. |
Iterable Interface
This is the top most interface in the collection. It has iterator(), forEach() and spliterator() methods.
Source code of Iterable:
public interface Iterable<T> {
/**
* Returns an iterator over elements of type {@code T}.
*
* @return an Iterator.
*/
Iterator<T> iterator();
// java 1.8 onwards
default void forEach(Consumer<? super T> action) {
Objects.requireNonNull(action);
for (T t : this) {
action.accept(t);
}
}
// Java 1.8 onwards
default Spliterator<T> spliterator() {
return Spliterators.spliteratorUnknownSize(iterator(), 0);
}
}
List Interface
List interface provides base of List data structure. It is further implemented by different list type of classes.
Example:
- List list = new ArrayList();
- List linkedList = new LinkedList();
- List vector = new Vector();
- List stack = new Stack();
ArrayList In Java
ArrayList is a class in java which implements List interface.
Example:
import java.util.*;
class ListExamples{
public static void main(String args[]){
//create list
ArrayList<String> list=new ArrayList<String>();
//add elemennts in list
list.add("Tom");
list.add("Bob");
Iterator itr=list.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output
Tom
Bob
LinkedList
Linked list is a class which implements List interface and provides features like a linked list data structure where each element is linked to another element.
Example:
import java.util.*;
class ListExamples{
public static void main(String args[]){
//create list
LinkedList<String> list=new LinkedList<String>();
//add elements in list
list.add("Tom");
list.add("Jerry");
Iterator itr=list.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output:
Tom
Jerry
Vector
It is similar to list but synchronized which means safe from threads but may become slow in terms of performance.
Example:
import java.util.*;
class MyCollections{
public static void main(String args[]){
//create vector
Vector<String> list=new Vector<String>();
//add elements in vector
list.add("Tom");
list.add("Bob");
Iterator itr=list.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output:
Tom
Bob
Stack
Stack is similar to list but it is LIFO type which means last in first out.
Example:
import java.util.*;
public class MyCollections{
public static void main(String args[]){
Stack stack = new Stack<String>();
stack.push("Tom");
stack.push("Rom");
String firstOut = stack.pop().toString();
System.out.println("Removed element: "+firstOut);
stack.push("Jerry");
Iterator<String> itr=stack.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
}
}
Output:
Removed element: Rom
Tom
Jerry
Queue Interface
Queue interface is similar to list but it maintains order of first in first out (FIFO), which means element which inserted first will be removed or go out first
Example:
Queue<String> pQueue = new PriorityQueue();
Queue<String> dQueue = new ArrayDeque();
Priority Queue
It compares the elements and does operations based on priority.
Example:
import java.util.*;
public class MyCollections{
public static void main(String args[]){
PriorityQueue<String> queue=new PriorityQueue<String>();
queue.add("A");
queue.add("B");
queue.add("C");
queue.add("E");
queue.add("D");
System.out.println("element:"+queue.element());
System.out.println("peek:"+queue.peek());
System.out.println("iterating the queue elements:");
Iterator itr=queue.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
queue.remove();
queue.poll();
System.out.println("after removing 1 elements:");
Iterator<String> itr2=queue.iterator();
while(itr2.hasNext()) {
System.out.println(itr2.next());
}
}
Output
element:A
peek:A
iterating the queue elements:
A
B
C
E
D
after removing 1 elements:
C
D
Deque Interface and ArrayDeque
Deque or doubly queue is a queue in which we can perform operations from both ends of the queue. ArrayDeque is an implementation of the Deque interface.
Example:
import java.util.*;
public class MyCollections2{
public static void main(String args[]){
Deque<String> queue=new ArrayDeque<>();
queue.add("D");
queue.add("B");
queue.add("C");
queue.add("E");
queue.add("A");
queue.addFirst("G"); // ADD at first
System.out.println("element:"+queue.element());
System.out.println("peek:"+queue.peek());
System.out.println("iterating the queue elements:");
Iterator itr=queue.iterator();
while(itr.hasNext()){
System.out.println(itr.next());
}
queue.remove(); //removes at first
queue.removeLast(); //removes last
queue.poll();
System.out.println("after removing 3 elements:");
Iterator<String> itr2=queue.iterator();
while(itr2.hasNext()) {
System.out.println(itr2.next());
}
}
}
Output
element:G
peek:G
iterating the queue elements:
G
D
B
C
E
A
after removing 2 elements:
B
C
E
Set Interface and HashSet
It provides structure of real life set data structure, HashSet and other implement Set interface, set contains unique value.
Set Example:
Set<data-type> hashSet = new HashSet<data-type>();
Set<data-type> linkedHashSet = new LinkedHashSet<data-type>();
Set<data-type> treeSet = new TreeSet<data-type>();
HasSet is a class which implements Set interface and contains unique values.
HashSet Example:
import java.util.*;
public class SetExample{
public static void main(String args[]){
Set<String> hashSet=new HashSet<String>();
hashSet.add("D");
hashSet.add("B");
hashSet.add("C");
hashSet.remove("C"); //removes a
System.out.println("after removing 3 elements:");
Iterator<String> itr=hashSet.iterator();
while(itr.hasNext()) {
System.out.println(itr.next());
}
}
}
Output
B
D
LinkedHashSet
LinkedHashSet is similar to LinkedList but an implementation of set.
Example:
import java.util.*;
public class SetExample{
public static void main(String args[]){
Set<String> hashSet=new LinkedHashSet<String>();
hashSet.add("D");
hashSet.add("B");
hashSet.add("C");
hashSet.remove("C"); //removes C
Iterator<String> itr=hashSet.iterator();
while(itr.hasNext()) {
System.out.println(itr.next());
}
}
}
Output
B
D
SortedSet Interface and TreeSet Class
SortedSet interface is similar to set interface, by default all elements are sorted in ascending(increasing) order.
TreeSet class implements SortedSet interface.
Example of SortedSet Interface
SortedSet<String> hashSet = new TreeSet<>();
Example:
import java.util.*;
public class SetExample{
public static void main(String args[]){
SortedSet<String> hashSet = new TreeSet<>();
hashSet.add("B");
hashSet.add("C");
hashSet.add("Z");
hashSet.add("A");
hashSet.add("A");
hashSet.remove("C"); //removes a
Iterator<String> itr=hashSet.iterator();
while(itr.hasNext()) {
System.out.println(itr.next());
}
}
}
Output
A
B
Z
Next Articles To Read
- Java ArrayList