Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Java Map Interface
Java Map is an interface which represents real world Map data structure, it provides methods to add, remove and do other useful operations on Map. Map stores elements in key, value pairs.
Important points related to Map.
- Map stores values in key, value pair.
- Keys are unique in a map.
- If we add duplicate key value pair, it will simplify update the previous values and won’t add any new element.
- Ideally a key should not be mutable.
- Map uses hashing algorithms/techniques to generate uniqueness from the key value to save the elements of map.
Map Hierarchy and Implementations
Map interface is implemented by HashMap, TreeHashMap and TreeMap classes.
PFB Map hierarchy diagram:
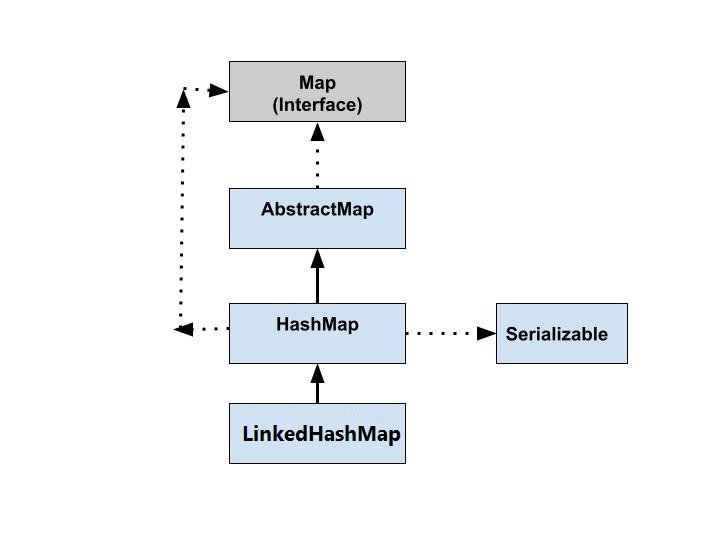
Below classes implement Map Interface:
· HashMap – No ordering maintained.
· LinkedHashMap – Insertion order maintained.
· TreeMap – Sorted ascending, order maintained.
Useful methods of Map interface
Method |
Description |
V put(Object key, Object value) |
Adds elements in the map. |
void putAll(Map map) |
Adds a map in another map. |
V putIfAbsent(K key, V value) |
Checks if an element is not present and then adds it. |
V remove(Object key) |
Removes an element of a given key. |
boolean remove(Object key, Object value) |
Removes specified key value pair. |
Set keySet() |
Returns keys of map in Set data type. |
Set<Map.Entry<K,V>> entrySet() |
It returns a set of key values. |
void clear() |
Clears map. |
V compute(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
|
V computeIfAbsent(K key, Function<? super K,? extends V> mappingFunction) |
|
V computeIfPresent(K key, BiFunction<? super K,? super V,? extends V> remappingFunction) |
|
boolean containsValue(Object value) |
Checks if an object is present or not. |
boolean containsKey(Object key) |
Checks if a key is present or not. |
boolean equals(Object o) |
Compares object’s values. |
void forEach(BiConsumer<? super K,? super V> action) |
Iterates. |
V get(Object key) |
Returns the object for the given key. |
V getOrDefault(Object key, V defaultValue) |
It returns an object for a given key or default value if not present. |
int hashCode() |
Returns hashCode value for element of Map. |
boolean isEmpty() |
Check if the map is Empty or not. |
V merge(K key, V value, BiFunction<? super V,? super V,? extends V> remappingFunction) |
|
V replace(K key, V value) |
Replaces value of specified key. |
boolean replace(K key, V oldValue, V newValue) |
Replace old value with new value. |
void replaceAll(BiFunction<? super K,? super V,? extends V> function) |
|
Collection values() |
Collection view of map. |
int size() |
Returns the size of the map. |
Map Example Non Generic
In a non-generic or old way, we don’t specify the data type of key and value.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;public class MapExamples {
public static void main(String[] args) {
Map myMap = new HashMap();
myMap.put("101", "Tom");
myMap.put("102", "Rom");
myMap.put("103", "Jonny");
myMap.get("101");Set eSet= myMap.entrySet();
Iterator itr = eSet.iterator();
while(itr.hasNext()) {
Map.Entry entry = (Map.Entry)itr.next();
System.out.println("Key:"+entry.getKey()+" value:"+entry.getValue());
}
}
}
Output
Key:101 value:Tom
Key:102 value:Rom
Key:103 value:Jonny
Map Example Generic
In generic map we specify the data type of key and value pair.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;public class MapExamples {
public static void main(String[] args) {Map<String, String> myMap = new HashMap<String, String>();
myMap.put("101", "Tom");
myMap.put("102", "Rom");
myMap.put("103", "Jonny");
myMap.get("101");
Set eSet= myMap.entrySet();Iterator itr = eSet.iterator();
while(itr.hasNext()) {
Map.Entry entry = (Map.Entry)itr.next();
System.out.println("Key:"+entry.getKey()+" value:"+entry.getValue());
}
}
}
Output
Key:101 value:Tom
Key:102 value:Rom
Key:103 value:Jonny
Java Map using comparingByKey() and comparingByValue methods for sorting and ordering
See below example where we have used comparingByKey and comparingByValue methods to sort the map with stream.
import java.util.Set;
import java.util.stream.Collectors;public class MapExamples {
public static void main(String[] args) {
Map<String, String> myMap = new HashMap<String, String>();
myMap.put("101", "Tom");
myMap.put("102", "Rom");
myMap.put("103", "Ann");
myMap.put("104", "Zin");
List listByKey = myMap.entrySet().stream()//Returns a stream of map
//Sorts based on provided comparator
.sorted(Map.Entry.comparingByKey(Comparator.reverseOrder())).collect(Collectors.toList());
System.out.println(listByKey);List<Map.Entry> listByValue = myMap.entrySet().stream()//Returns a stream of map
//Sorts based on provided comparator
.sorted(Map.Entry.comparingByValue(Comparator.naturalOrder())).collect(Collectors.toList());
System.out.println(listByValue);
}
}
Output
[104=Zin, 103=Ann, 102=Rom, 101=Tom]
[103=Ann, 102=Rom, 101=Tom, 104=Zin]
Next Article
- HashMap