Core JAVA
Java Runtime Environment (JRE)
Java Virtual Machine (JVM)
Java overview
Java basics
Java Objects and classes
Java Constructors
Java basic datatypes
Java variable types
Java modifiers/Access Modifiers In Java
Java Basic Operators
Java Loops and Controls
Java conditions
Java numbers and characters
Java strings
Java arrays
Java date time
Java methods
Java file and IO operations
Java exceptions
Inner class
Java OOPs Concepts
Java Inheritance
Java Polymorphism
Java Abstraction
Java Encapsulation
Java Interface
Cohesion and Coupling
Association, Aggregation and Composition
Java Collections
Java ArrayList
Java LinkedList
Set and HashSet
LinkedHashSet and TreeSet
Queue and PriorityQueue
Deque and PriorityQueue
Java Map Interface
Java HashMap
Internal Working Of Java HashMap
Java Mutithread
Methods of Thread In Java
Join , run & Start Method in Threads
Difference b/w start & run Methods in Threads
Java Concurrency Package & its Features
CountDownLatch, CyclicBarrier, Semaphore and Mutex in Thread
Java Polymorphism
Polymorphism means ability to take multiple forms, we can break polymorphism into two parts poly and morphism where poly means multiple and morphism means form. In OOP methods in a class may look same by name but can perform different action which will be called polymorphism. In this article we look in detail on java polymorphism.
For example Student class can be extended by ArtsStudent and MathsStudent child class and may have same method in all three but actions inside methods will be different this will be a simple example of polymorphism in java.
Example:
class Student { public void displayStudent(){ System.out.println("I am student class"); } } class ArtsStudent extends Student { @Override public void displayStudent(){ System.out.println("I am arts student class"); } } class MathsStudent extends Student { @Override public void displayStudent(){ System.out.println("I am maths student class"); } } class Demo{ public static void main(String[] args) { //Demo of runtime polymorphism in java Student student = new Student(); Student artsStudent = new ArtsStudent(); Student mathsStudent = new MathsStudent(); student.displayStudent(); artsStudent.displayStudent(); mathsStudent.displayStudent(); } }
Output:
I am student class
I am arts student class
I am maths student class
Types Of Java Polymorphism
There are two types of polymorphism in java as mentioned below;
- Compile Time Polymorphism – It is achieved while compile time.
- Runtime Polymorphism – It is achieved at run time.
Compile Time Polymorphism (Overloading)
Compile time polymorphism is achieved at code creation and compilation, if we have more than one methods with same name but different arguments in a class then it will be called compile time polymorphism.
Example:
In below example we create Operations class with method operate() with multiple definitions and arguments.
class Operator{ public void operate() { System.out.println("I am no argument operator"); } public void operate(int a) { System.out.println("I am single argument operator, value of a is:"+a); } public void operate(int a, int b) { System.out.println("I am two arguments operator, value of a is: "+a+" and b is: "+b); } public static void main(String[] args) { Operator operator = new Operator(); operator.operate(); operator.operate(10); operator.operate(10, 20); } }
Output:
I am no argument operator
I am single argument operator, value of a is:10
I am two arguments operator, value of a is: 10 and b is: 20
Runtime Polymorphism (Overriding)
Runtime polymorphism is achieved at execution or run time, if we have a class and a child class extends it and overrides its method and at runtime, if objects of child and parent created and assigned to parent type and common method called in both, then at run time it will determine which of class’s method (parent or child) to call and execute, this is called runtime polymorphism.
Example:
In below example Car class is inherited by PetrolCar and ElectricCar and displayCarType() method is available in all three classes, so when we call with help of car’s object it executes displayCarType() method of Car class and when we call same method with instance of PetrolCar will call displayCarType() of PetrolCar and same is with ElectricCar class.
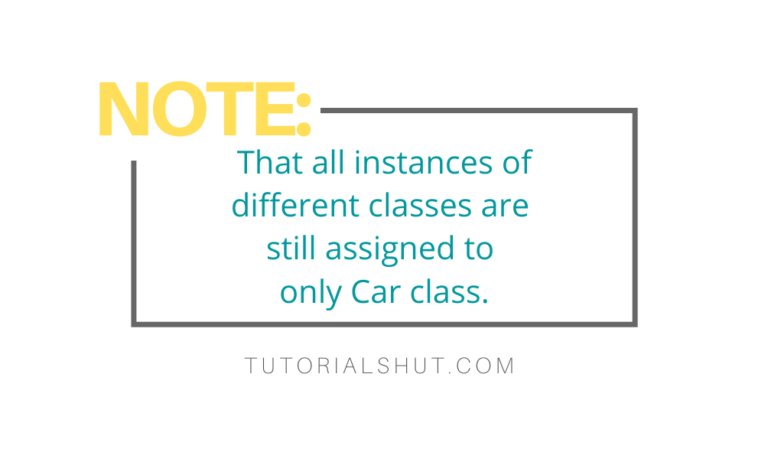
class Car{ public void displayCarType() { System.out.println("I am common car type."); } } class PetrolCar extends Car { @Override public void displayCarType() { System.out.println("I am PetrolCar."); } } class ElectricCar extends Car { @Override public void displayCarType() { System.out.println("I am ElectricCar."); } class DemoRunTimePolymorphisam{ public static void main(String[] args) { Car car = new Car(); Car petrolCar = new PetrolCar(); Car eCar = new ElectricCar(); car.displayCarType(); petrolCar.displayCarType(); eCar.displayCarType(); } }
Output:
I am common car type.
I am PetrolCar.
I am ElectricCar.
References
Reference article from Oracle:Â PolymorphismÂ