Cypress tutorials
what is cypress?
cypress architecture
Selenium & cypress Architecture
cypress features
Cypress Installation and project setup
node-js
Visual Studio Code
Create New Project in Cypress
Open and start Cypress
cypress executable location
cypress open
Switch browser in cypress
Run TestCase in cypress
cypress Test Runner
cypress command line
Basic Test Case in Cypress
Mocha test framework in cypress
Describe and it in cypress
Basic test case
Hooks in Cypress
before() Hooks in Cypress
beforeEach() Hooks in Cypress
after() Hooks in Cypress
afterEach() Hooks in Cypress
Default assertion in cypress
When not to assert in cypress
How to modify default assertion timeout
Assertions in cypress
Implicit assertion using should & and
How to use should & and for assertion
Explicit assertion using should & and
How to use expect in assertion
Common assertions in cypress
Basic Comands in cypress
Custom Comands in cypress
Custom command syntax & command.js
Parent custom commands in cypress
Child custom commands in cypress
Dual custom commands in cypress
Example of cypress custom commands
Variables & Aliases in cypress
Return Values In Cypress
Closures in cypress
Hooks in Cypress
In Cypress, hooks are functions that are executed at specific points during the test lifecycle. They allow you to perform setup and teardown operations, and to modify the behavior of Cypress commands and assertions.These are helpful to set conditions that you want to run before a set of tests or before each test.
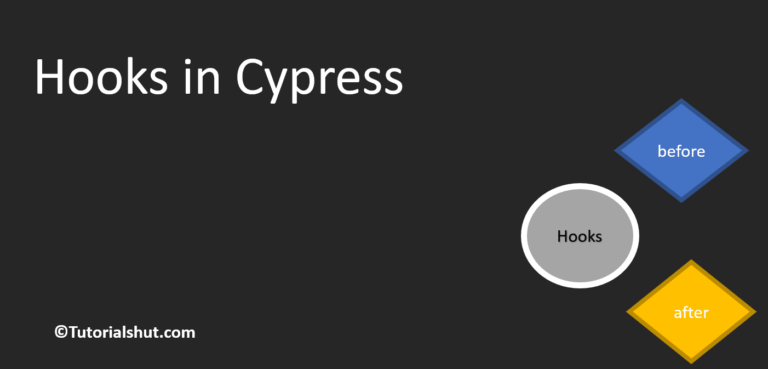
Some commonly used hooks in cypress are:
We will go through each one of them with examples one by one.
before() hook in Cypress
before() – runs once before all tests in a describe block.
before() block is called once before all tests in a file. You can use this function to set up any global state or perform any necessary setup that only needs to be done once. Example:
describe('Login tests', () => { before(() => { // runs once before all tests in this block cy.visit('/login') }) it('should display the login form', () => { // test code }) // more tests... })
beforEach() hooks in Cypress
beforeEach() – runs before each test in a describe block. beforeEach() block is called before each test in a file. You can use this function to set up any state or perform any setup that needs to be done before each test. For example, you might use this function to log in to your application or navigate to a specific page. Example:describe('Shopping cart tests', () => { beforeEach(() => { // runs before each test in this block cy.visit('/cart') }) it('should display the cart items', () => { // test code }) // more tests... })
after() hooks in Cypress
after() – runs once after all tests in a describe block.
after() block is called once after all tests in a file have completed. You can use this function to perform any final cleanup or teardown that needs to be done. Example:
describe('Logout tests', () => { after(() => { // runs once after all tests in this block cy.logout() }) it('should log out the user', () => { // test code }) // more tests... })
afterEach() hooks in Cypress
afterEach() – runs after each test in a describe block.
afterEach() block is called after each test in a file. You can use this function to clean up any state or perform any teardown that needs to be done after each test. For example, you might use this function to log out of your application or reset any modified data.
describe('Search tests', () => { afterEach(() => { // runs after each test in this block cy.clearSearchResults() }) it('should display search results for a valid query', () => { // test code }) it('should display no results for an invalid query', () => { // test code })// more tests… })
Use the beforeAll() and afterAll() hooks to run setup and teardown operations at the beginning and end of the entire test suite, rather than just within a specific describe block.
Complete example is below:
describe('My Test Suite', () => { before(() => { // Perform global setup here }) beforeEach(() => { // Perform setup before each test here }) it('TestCase 1', () => { // Test 1 code here }) it('TestCase 2', () => { // Test 2 code here }) afterEach(() => { // Perform teardown after each test here }) after(() => { // Perform final teardown here }) })
In above example, the before() hook is used to perform global setup, the beforeEach() hook is used to perform setup before each test, the afterEach() hook is used to perform teardown after each test, and the after() hook is used to perform final teardown. The it() function is used to define individual tests.