Selenium WebDriver
Selenium Introduction
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
Difference between findElement and findElements Commands with examples
This article will present you with a complete idea about findElement() and findElements() command in selenium WebDriver with examples
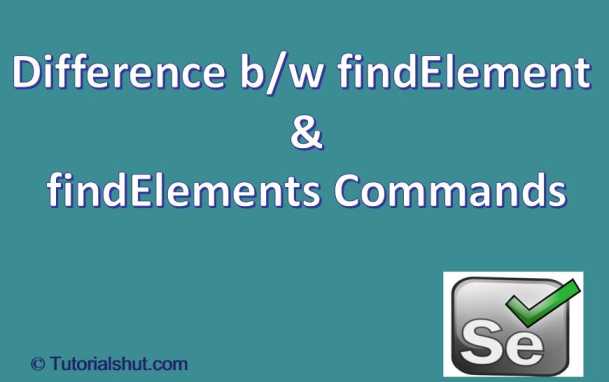
We will learn below topics in this article
findElement() method
- findElement() command is used to find a single element on the web page.
- This command returns the object of the first matching element.
- When element is not found using findElement() command , it will throw the exception ‘NoSuchElementException’
- There are several ways for identifying a web element within the web page for e.g. ID, Name, Class Name, Link Text, Partial Link Text, Tag Name and XPATH.
Syntax-
WebElement element = driver.findElement(By.LocatorStrategy<br />(“Locator Value”));
Locator Strategy can be the following values.
- ID
- Name
- Class Name
- Tag Name
- Link Text
- Xpath
Locator Strategy | Syntax | Description |
By ID | driver.findElement(By.id()) | Locate Element using ID |
By Name | driver.findElement(By.name()) | Locate Element using Name |
By Xpath | driver.findElement(By.xpath ()) | Locates Element using XPath |
By CSS | driver.findElement(By.cssSelector()) | Locate Element using css selector |
By Tag Name | driver.findElement(By.tagName(<tagname)) | Locate Element using html Tag name |
By Link Text | driver.findElement(By.linkText()) | Locate Element using link text |
By Patial Link text | driver.findElement(By.partialLinkText()) | Locate Element using Partial link text |
By Class Name | driver.findElement(By.className()) | Locate Element using Class Name |
findElements() method.
- findElements() command is used to identify the list of web elements in the web page.
- Return type of this method is the list of all the elements matching within the Page
- If None of the elements is matching then this method will return an empty List.
Syntax-
List links =driver.findElements(By.xpath(“//table/tr”));
How to use Selenium findElement Command ?
Scenario:
-
- Open the browser
- Navigate to https://tutorialshut.com/demo-website-for-selenium-automation-practice/
- Navigate to section “Sum of two numbers ‘A’ & ‘B’ (Input Form)”
- Find and enter values of A and B
- find element and click ‘Get Sum’ button

Code Implementation
package com.test; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class DemoFindElement { public static WebDriver driver; public static void main(String[] args) throws InterruptedException { // Create a new instance of the Chrome driver System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Launch the URL driver.get("https://tutorialshut.com/demo-website-for-selenium-automation-practice/"); //Maximize the window driver.manage().window().maximize(); // find element using ID and enter value driver.findElement(By.id("num1")).sendKeys("1"); driver.findElement(By.id("num2")).sendKeys("2"); // find element using Xpath and click driver.findElement(By.xpath("//button[text()='Get Sum']")).click(); // close the driver driver.close(); } }
How to use Selenium findElements Command?
Scenario
-
- Open the browser
- Navigate to https://tutorialshut.com/demo-website-for-selenium-automation-practice/
- Find the text of radio buttons and print on console.

Code Implementation
package com.test; import java.util.List; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.WebElement; import org.openqa.selenium.chrome.ChromeDriver; public class DemoFindElements { public static WebDriver driver; public static void main(String[] args) throws InterruptedException { // Create a new instance of the Chrome driver System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); WebDriver driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Launch the URL driver.get("https://tutorialshut.com/demo-website-for-selenium-automation-practice/"); // Maximize the window driver.manage().window().maximize(); // find elements for Radio button using Name List elements = driver.findElements(By.name("gender")); System.out.println("Number of elements:" + elements.size()); for (int i = 0; i < elements.size(); i++) { System.out.println("Radio button text:" + elements.get(i).getAttribute("value")); } // close the driver driver.close(); } }
Selenium WebDriver Tutorials
Selenium Introduction
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report