Selenium WebDriver
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
Read and Write csv file in Selenium
This Tutorial represent a complete idea about how to read and write test data/result in csv file in selenium automation.
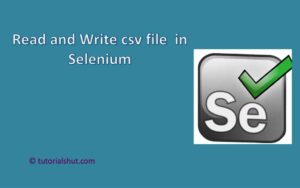
What is CSV file ?
A comma-separated values (CSV) file is a delimited text file that uses a comma to separate values.
Each line of the file is a data record. Each record consists of one or more fields, separated by commas.
We can handle the CSV file using the below packages :
- Apache Commons CSV
- Open CSV
Below are the steps used to integrate selenium project with Apache Commons CSV
If Maven Project is used for automation then we have to add below dependency in pom.xml file
<dependency>Â Â Â
<groupId>org.apache.commons</groupId>Â Â Â
<artifactId>commons-csv</artifactId>Â Â Â
<version>1.5</version>
</dependency>
And if Generic automation project is used then jar file can be downloaded from below location
https://commons.apache.org/proper/commons-csv/download_csv.cgi
We can read the CSV file using Apache Commons CSV library ; there could be two kinds of CSV files.
- CSV file with Header
- CSV file without Header
How to read csv file without header with Apache Commons CSV?
 Open Notepad and enter below values in format mention below and Save file as  .csv format
Ram,Kumar,Infosys
Shyam,Gahlot,TCS
Denial,W,Wipro
Steps:
- Create the CSV file.
- Create a new BufferReader object and pass the path of CSV file
- Parse the BufferReader object into comma-separated values so create an object for CSV parser and passing reader reference(whatever going to parse), and the delimiter for parsing
- Now iterate each row and column as data is loaded in csvParse object.
//Code Snippet
package com.test; import org.testng.annotations.Test; import org.apache.commons.csv.CSVFormat; import org.apache.commons.csv.CSVParser; import org.apache.commons.csv.CSVRecord; import java.io.IOException; import java.io.Reader; import java.nio.file.Files; import java.nio.file.Paths; public class CSVReader { @Test public void readCSV() throws IOException { String path = "C:\\File\\abc.csv"; // Create a new BufferReader object and pass the path of CSV file Reader reader = Files.newBufferedReader(Paths.get(path)); // parse the file into csv values CSVParser parse = new CSVParser(reader, CSVFormat.DEFAULT); for (CSVRecord csvRecord : parse) { // Accessing Values by Column Index String firstname = csvRecord.get(0); String lastName = csvRecord.get(1); String company = csvRecord.get(2); // print the value to console System.out.println("Record No - " + csvRecord.getRecordNumber()); System.out.println("FirstName : " + firstname); System.out.println("Last Name : " + lastName); System.out.println("Company name : " + company); } } }
How to read csv file with header with Apache Commons CSV?
Open Notepad and enter below values in format mention below and Save file as  .csv format
FirstName, LastName, CompanyName
Ram,Kumar,Infosys
Shyam,Gahlot,TCS
Denial,W,Wipro
Below is the complete program for reading .csv file
package com.test; import org.testng.annotations.Test; import org.apache.commons.csv.CSVFormat; import org.apache.commons.csv.CSVParser; import org.apache.commons.csv.CSVRecord; import java.io.IOException; import java.io.Reader; import java.nio.file.Files; import java.nio.file.Paths; public class CSVReader { @Test public void readCSV() throws IOException { String path = "C:\\File\\abc.csv"; // read the file Reader reader = Files.newBufferedReader(Paths.get(path)); CSVParser csvParser = new CSVParser(reader, CSVFormat.DEFAULT .withHeader("FirstName", "LastName", "CompanyName") .withIgnoreHeaderCase() .withTrim()); for (CSVRecord csvRecord : csvParser) { // Accessing values by the names assigned to each column String fname = csvRecord.get("FirstName"); String lname = csvRecord.get("LastName"); String company = csvRecord.get("CompanyName"); System.out.println("Record No - " + csvRecord.getRecordNumber()); System.out.println("FirstName : " + fname); System.out.println("LastName : " + lname); System.out.println("CompanyName : " + company); } } }
Recommended Articles Â
Selenium WebDriver Tutorials
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report