Selenium WebDriver
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
How to launch browser using Selenium WebDriver ?
This article will present you with a complete idea about How to launch different browsers using selenium commands.
We will learn below topics in this article
Introduction of Browser launch Command
- Selenium WebDriver calls the native methods of the different browsers to automate them.
- Different browsers have different drivers which are used to automate for example FirefoxDriver for Firefox browser, ChromeDriver for Google Chrome, InternetExplorerDriver for Internet Explorer etc.
For example for Launching Firefox browser below command is used
WebDriver driver = new FirefoxDriver();
Meaning of WebDriver driver = new FirefoxDriver();
Here, ‘WebDriver’ is an interface and we are creating a reference variable ‘driver’ of type WebDriver, instantiated using the ‘FireFoxDriver’ class.All the methods which are declared in webDriver interface are implemented by FirefoxDriver class.
So creating a reference variable of type WebDriver assigns the driver object to different browser specific drivers.
We can also create a reference variable of type FirefoxDriver like this-
FirefoxDriver driver = new FirefoxDriver();
Using the above command FirefoxDriver instance will get created and then we can use and act on methods implemented by FirefoxDriver and supported by Firefox Browser only.
So when we will use other browsers for example at the the multi browser testing then we have to specifically create individual objects as below :
InternetExplorerDriver driver = new InternetExplorerDriver(); ChromeDriver driver = new ChromeDriver();
So if we use WebDriver driver then we can use the already initialised driver for all browsers we want to automate e.g. Mozilla, Chrome, InternetExplorer, Safari etc.
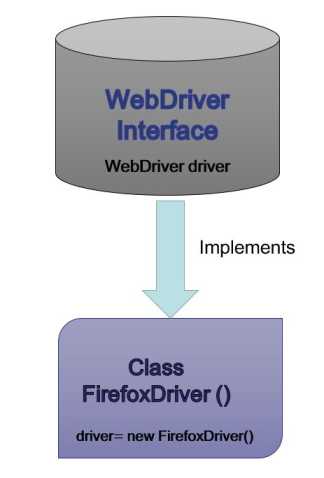
Launch Firefox Browser
Below are the steps required to launch the Firefox browser.
-
- Download geckodriver.exe from here specific to your OS and save it somewhere on your computer.
- Set the System Property for “webdriver.gecko.driver” with the geckodriver.exe path – System.setProperty(“webdriver.gecko.driver”,”geckodriver.exe path”);
package com.test; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; public class LaunchFirefoxBrowser { public static void main(String[] args) { // Creating a driver object WebDriver driver; // Setting webdriver.gecko.driver property System.setProperty("webdriver.gecko.driver", pathToGeckoDriver + "\\geckodriver.exe"); // Instantiating driver object and launching browser driver = new FirefoxDriver(); // Using get() method to open a webpage driver.get("https://tutorialshut.com"); // Closing the browser driver.quit(); } }
Launch Internet Explorer Browser
Below are the steps required to launch the Internet Explorer browser.
-
- Download IEDriverServer.exe from here and save it somewhere on your computer.
- Set the System Property for “webdriver.ie.driver” with the IEDriverServer.exe path – System.setProperty(“webdriver.ie.driver”,”IEDriverServer.exe path”);
package com.test; import org.openqa.selenium.WebDriver; import org.openqa.selenium.ie.InternetExplorerDriver; public class LaunchIEBrowser { public static void main(String[] args) { // Creating a driver object WebDriver driver; // Setting webdriver.ie.driver property System.setProperty("webdriver.ie.driver", pathToIEDriver + "\\IEDriverServer.exe"); // Instantiating driver object and launching browser driver = new InternetExplorerDriver(); // Using get() method to open a webpage driver.get("https://tutorialshut.com"); // Closing the browser driver.quit(); } }
Launch Chrome Browser
Below are the steps required to launch the Chrome browser.
-
- Download chromeDriver.exe from here and save it somewhere on your computer.
- Set the System Property for “webdriver.chrome.driver” with the chromeDriver.exe path – System.setProperty(“webdriver.ie.driver”,”chromeDriver.exe path”);
package com.test; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class LaunchChromeBrowser { public static void main(String[] args) { // Creating a driver object WebDriver driver; // Setting webdriver.chrome.driver property System.setProperty("webdriver.chrome.driver", pathToChromeDriver + "\\chromeDriver.exe"); // Instantiating driver object and launching browser driver = new ChromeDriver(); // Using get() method to open a webpage driver.get("https://tutorialshut.com"); // Closing the browser driver.quit(); } }
Selenium WebDriver Tutorials
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report