Selenium WebDriver
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
How to Handle iframes using Selenium WebDriver ?
This article will present you with a complete idea about handle iframes using Selenium WebDriver. iFrame in Selenium Webdriver is a web page or an inline frame ,which is embedded in another web page or an HTML document .
We will learn below topics in this article
- What is an iframe?
- How to Identify a Frame on a Page using Selenium?
- How to find total number of iFrames on a webpage in Selenium WebDriver?
- How to switch to Frames by Index in Selenium WebDriver?
- How to switch to Frames by Name in Selenium ?
- How to switch to Frame by ID in Selenium ?
- How to switch to Frame by WebElement in Selenium WebDriver?
- How to switch Back to Main Frame in Selenium WebDriver?
What is an iframe?
- The <iframe> tag defines an inline frame.
- So An inline frame is used to embed another html document within the current HTML document.
- An iframe tag is defined <iframe></iframe> tags.
Below is the example which shows two inline frame/ document within the current page.
<html>
<body>
<h1>iframe Example</h1>
<iframe src="https://tutorialshut.com/" width="100%" height="300">
</iframe>
<iframe src="https://tutorialshut.com/" width="100%" height="300">
</iframe>
</body>
</html>
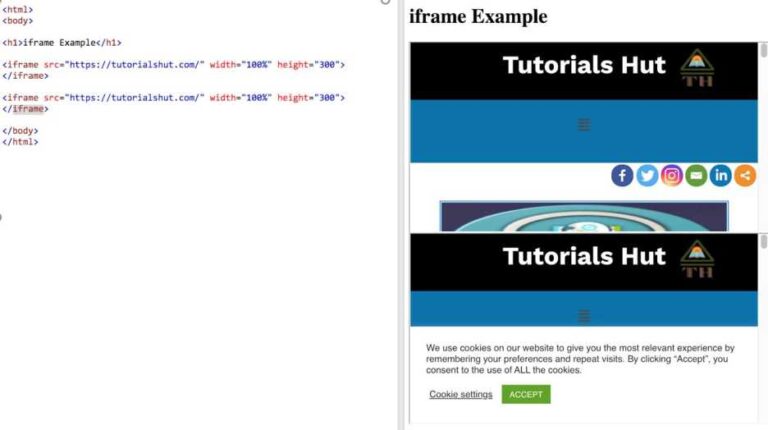
How to Identify a Frame on a Page?
Right-click on the element and If you find an option like This Frame, view Frame source then it shows the page includes frames below is the example-

Second method to find the iframe is , Right-click on the page and click on View Page Source ,Search ‘iframe’ tag and if it is mound , means the page has iframes.
How to find total number of iFrames on a webpage?
Below are few approaches used to find iframes on a web page
1)Using tag name
List framesList =driver.findElements(By.tagName("iframe")).size() int numOfFrames = framesList.size();
2) using xpath
List framesList = driver.findElements(By.xpath("//iframe")); int numOfFrames = frameList.size();
How to switch to Frames by Index?
Lets say A webpage consists 2 iframes then index will start from 0 and then 1 respectively.
so first iframe has index 0 and second iframe has 1
Syntax –
driver.switchTo().frame(int index);
Below example shows how to switch to first iframe
public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); driver = new ChromeDriver(); driver.get("URL which has iframe"); //Switch to first iframe using index driver.switchTo().frame(0); driver.close(); }
How to switch to Frames by Name
if you look at the html code for iFrame in the web page then sometimes you can find the name attribute. We can switch to that iFrame using the name. Below is the syntax for this.
Syntax:
driver.switchTo().frame(“framename”);
Example-
public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); driver = new ChromeDriver(); driver.get("URL which has iframe"); //Switch to first iframe using name driver.switchTo().frame(“name attribute of iframe”); driver.close(); }
How to switch to Frame by ID
IF iframe consist Id attribute by looking at the html of Iframe then We can switch to that iFrame using the id .Below is the syntax for this.
Syntax:
driver.switchTo().frame(“id”);
Example:
public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); driver = new ChromeDriver(); driver.get("URL which has iframe"); //Switch to first iframe using ID driver.switchTo().frame(“id attribute of iframe”); driver.close(); }
How to switch to Frame by WebElement
We can switch to an iframe by finding an element using any of the locator strategies and then passing it to the switchTo command.
Syntax:
driver.switchTo().frame(“webElement”);
Example:
public static void main(String[] args) { System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); driver = new ChromeDriver(); driver.get("URL which has iframe"); //Switch to first iframe using WebElement driver.switchTo().frame(“iframe webElement”); driver.close(); }
How to switch Back to Main Frame
Suppose a main web page consists of two iframes and we have switched into one of the inner iframes .
Now if we want to switch back to main web page or parent page then we can switch to main page using below command
Syntax-
driver.switchTo().defaultContent(). OR driver.switchTo().parentFrame():

SCENARIO
Let’s consider a web page which has two iframes , one is the outer iframe and other iframe is inside the outer frame.
- Open chrome browser
- Navigate to URL containing iframes
- Find total number of frames in the web page
- Switching the Outer Frame and print the text inside outer frame
- Find and print the total number of frames inside outer frame
- Switch to inner frame and print the text inside outer frame
- Switch back to the outer frame
- Close the browser
Code implementation
package com.test; import java.util.concurrent.TimeUnit; import org.openqa.selenium.By; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; public class HandleFrames { public static WebDriver driver; public static void main(String[] args) throws InterruptedException { // Create a new instance of the Chrome driver System.setProperty("webdriver.chrome.driver", "D:\\Drivers\\chromedriver.exe"); driver = new ChromeDriver(); driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS); // Launch the URL driver.get("URL containing iframes"); driver.manage().timeouts().implicitlyWait(2, TimeUnit.SECONDS); int size = driver.findElements(By.tagName("iframe")).size(); // prints the total number of frames in the web page System.out.println("Total no of Frames --" + size); // Switching the Outer Frame driver.switchTo().frame(0); // Printing the text in outer frame System.out.println(driver.findElement(By.xpath("xpath of the outer element ")).getText()); // prints the total number of frames inside outer frame size = driver.findElements(By.tagName("iframe")).size(); System.out.println("Total Frames --" + size); driver.switchTo().frame(0); // Switching to inner frame // Printing the text in inner frame System.out.println(driver.findElement(By.xpath("xpath of the inner element ")).getText()); driver.switchTo().defaultContent(); driver.close(); } }
Selenium WebDriver Tutorials
Benefits of Selenium
Four components of Selenium
Difference b/w Selenium IDE, RC & WebDriver
Selenium WebDriver Architecture
Background when user execute selenium code
Download and Install Java
Download and Install Eclipse
Download Selenium WebDriver
Selenium WebDriver Locators
Selenium - Launch Browser
Selenium WebDriver Waits
Selenium- Implicit wait
Selenium- Explicit wait
Selenium- Fluent wait
Selenium- Commonly used commands
Selenium- findElement & findElements
Selenium- Selenium-Handling check Box
Selenium- Handling Radio button
Selenium- Handling drop down
Selenium- Take Screenshot
Selenium- Handle Web Alerts
Selenium- Multiple Windows Handling
Selenium- Handle iframes
Selenium- Upload a file
Selenium- Download a file
Selenium- Actions Class Utilities
Selenium- Mouse Actions
Selenium- Keyboards Events
Selenium- Handle mouse hover Actions
Selenium- Drag and Drop
Selenium- Scroll a WebPage
Selenium- Context Click / Right Click
Selenium- Double Click
Selenium- Desired Capabilities
Selenium- Assertions
Selenium- Exceptions and Exception Handling
Selenium- Difference b/w driver.close() & driver.quit()
Selenium- difference b/w driver.get() & driver.navigate()
Selenium- JavascriptExecutor
Selenium- Read excel file using Fillo API
Selenium- Database Testing using Selenium
Selenium- Read & write excel file using Apache POI
Selenium- Read and Write csv file in Selenium
Selenium- Dynamic Web Table Handling
Selenium- Maven Integration with Selenium
Selenium- Set up Logging using Log4j
Selenium-Implement Extent Report
About Author
Nitesh is working as a Quality Analyst with more than 10 years of comprehensive experience in test automation, performance testing , DevOps etc .He likes to share his wealth of knowledge across software test Process